Welcome back to HiGiBuGi. Argentina qualified for the round of 16 and in today's blog, I will
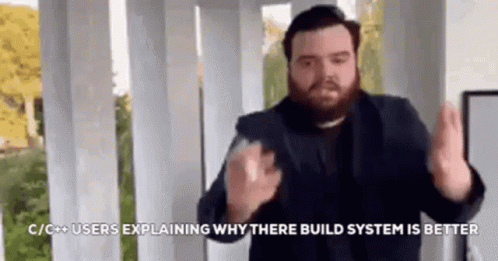
show everyone how my genetic heritage
has naturally gifted me with tech skills. Without wasting any time, I'll hop onto the subject matter.
ARDUINO PROGRAMMING
There are 4 tasks that will be explained in this page:
1. Input devices:
a. Interface a potentiometer analog input to maker UNO board and measure/show its signal in serial monitor Arduino IDE.
b. Interface a LDR to maker UNO board and measure/show its signal in serial monitor Arduino IDE
2. Output devices:
a. Interface 3 LEDs (Red, Yellow, Green) to maker UNO board and program it to perform something (fade or flash etc)
b. Include the pushbutton on the MakerUno board to start/stop part 2.a. above
For each of the tasks, I will describe:
The program/code that I have used and explanation of the code. The code is in writable format (not an image).
The sources/references that I used to write the code/program.
The problems I encountered and how I fixed them.
The evidence that the code/program worked in the form of video of the executed program/code.
My Learning reflection on the overall Arduino programming activities.
Input devices: Interface a potentiometer analog input to maker UNO board and measure/show its signal in serial monitor Arduino IDE.
1. Below are the code/program I have used and the explanation of the code.
Code/program in writeable format | Explanation of the code |
int sensorPin = A0; int ledPin = 13; int sensorValue = 0; void setup() { pinMode(ledPin,OUTPUT); } void loop() { sensorValue = analogRead(sensorPin); digitalWrite(ledPin, HIGH); delay(sensorValue); digitalWrite(ledPin, LOW); delay(sensorValue); } | sensorPin is initialised to be A0 ledPin is intialised to be PIN 13 sensorValue is initialised to be 0 Within voidsetup() pinMode(ledPin,OUTPUT) : Establishes PIN 13 as the output Within voidloop() sensorValue = analogRead(sensorPin) : reads the value of A0 and writes it into an integer variable digitalWrite(ledPin, HIGH) : Turns on LED 13 using a digital function delay(sensorValue) : delay function changes as the knob of the potentiometer turns. digitalWrite(ledPin, LOW) : Turns off LED 13 using a digital function |
2. Below are the hyperlink to the sources/references that I used to write the code/program.
Hyperlink
3. Below are the problems I have encountered and how I fixed them.
I honestly didn't find any problems when attempting this task as I just followed the diagrams provided for the breadboard wiring and copy-pasted the code into ArduinoIDE
4. Below is the short video as evidence that the code/program works.
Input devices: Interface a LDR to maker UNO board and measure/show its signal in serial monitor Arduino IDE:
1. Below are the code/program I have used and the explanation of the code.
Code/program in writeable format | Explanation of the code |
int LDR = A0; int ledPin = 13; int LDRvalue = 0; void setup() { pinMode(ledPin,OUTPUT); } void loop() { LDRvalue = analogRead(LDR); if(LDRvalue > 600) digitalWrite(ledPin, HIGH); else digitalWrite(ledPin, LOW); } | LDR is initialised to be A0 ledPin is intialised to be PIN 13 LDRValue is initialised to be 0 Within void setup() pinMode(ledPin,OUTPUT) : Establishes PIN 13 as the output Within void loop() LDRValue = analogRead(LDR) : reads the value of A0 and writes it into an integer variable, light. digitalWrite(ledPin, HIGH) : Turns on LED 13 using a digital function if (LDRvalue > 600) digitalWrite(ledPin, LOW) : Turns off LED 13 using a digital function if (LDRvalue < 600) |
2. Below are the hyperlink to the sources/references that I used to write the code/program.
3. Below are the problems I have encountered and how I fixed them.
When I made my circuit, I initially thought that when light shines on the LDR, the LED would light up. So when it did the complete opposite, I was awed. However, when looking at this problem as a lamp post; when the surroundings get darker, the LDR on top of the lamp post would detect the lack of light causing the lamp post to turn on. So in actuality, My programme and circuit did work as expected.
4. Below is the short video as the evidence that the code/program work.
Output devices: Interface 5 LEDs (Red, Yellow, Green) to maker UNO board and program it to perform something (fade or flash etc)
1. Below are the code/program I have used and the explanation of the code.
Code/program in writeable format | Explanation of the code |
const int greenLedVehicle = 5;
const int yellowLedVehicle = 6;
const int redLedVehicle = 7;
const int greenLedPedestrian = 3;
const int redLedPedestrian = 4;
void setup()
{
pinMode(greenLedVehicle, OUTPUT);
pinMode(yellowLedVehicle, OUTPUT);
pinMode(redLedVehicle, OUTPUT);
pinMode(greenLedPedestrian, OUTPUT);
pinMode(redLedPedestrian, OUTPUT);
digitalWrite(greenLedVehicle, HIGH);
digitalWrite(redLedPedestrian, HIGH);
}
void loop()
{
digitalWrite(greenLedVehicle, LOW);
digitalWrite(yellowLedVehicle, HIGH);
delay(2000);
digitalWrite(yellowLedVehicle, LOW);
digitalWrite(redLedVehicle, HIGH);
delay(1000);
digitalWrite(redLedPedestrian, LOW);
digitalWrite(greenLedPedestrian, HIGH);
delay(5000);
digitalWrite(greenLedPedestrian, LOW);
digitalWrite(redLedPedestrian, HIGH);
delay(1000);
digitalWrite(redLedVehicle, LOW);
digitalWrite(greenLedVehicle, HIGH);
delay(2000);
}
| greenLedVehicle is initialised to be PIN 5 yellowLedVehicle is initialised to be PIN 6 redLedVehicle is initialised to be PIN 7 greenLedPedestrian is initialised to be PIN 3 redLedPedestrian is initialised to be PIN 4 Within void setup() pinMode(greenLedVehicle, OUTPUT); pinMode(yellowLedVehicle, OUTPUT); pinMode(redLedVehicle, OUTPUT); pinMode(greenLedPedestrian, OUTPUT); pinMode(redLedPedestrian, OUTPUT); : Establishes PINs 3, 4, 5, 6, 7 as output digitalWrite(greenLedVehicle, HIGH); digitalWrite(redLedPedestrian, HIGH); : PINs 4 and 5 are always on before pushing the pushButton Within voidloop() digitalWrite(greenLedVehicle, LOW); digitalWrite(yellowLedVehicle, HIGH); delay(2000); : PIN 5 turns off, PIN 6 turns on and there will be a 2-second delay digitalWrite(yellowLedVehicle, LOW); digitalWrite(redLedVehicle, HIGH); delay(1000); : PIN 6 turns off, PIN 7 turns on and there will be a 1-second delay digitalWrite(redLedPedestrian, LOW); digitalWrite(greenLedPedestrian, HIGH); delay(5000); : PIN 4 turns off, PIN 3 turns on and there will be a 5-second delay digitalWrite(greenLedPedestrian, LOW); digitalWrite(redLedPedestrian, HIGH); delay(1000); : PIN 3 turns off, PIN 4 turns on and there will be a 1-second delay digitalWrite(redLedVehicle, LOW); digitalWrite(greenLedVehicle, HIGH); delay(2000); : PIN 7 turns off, PIN 5 turns on and there will be a 2-second delay before the loop resets
|
2. Below are the hyperlink to the sources/references that I used to write the code/program.
Hyperlink
3. Below are the problems I have encountered and how I fixed them.
Since I wanted to insert a fading feature to the lights when each of them turned on, I found it difficult to insert the fading code into the original code that I found online. The original code also came with the pushbutton feature, so I tried to carefully remove the pushButton code so that the circuit would always be working without the need for the button. However, after multiple attempts of trial and error, I finally got it to work and it personally looks amazing to me.
4. Below is the short video as the evidence that the code/program work.
Output devices: Include pushbutton to start/stop the previous task
1. Below are the code/program I have used and the explanation of the code.
Code/program in writeable format | Explanation of the code |
const int greenLedVehicle = 5; const int yellowLedVehicle = 6; const int redLedVehicle = 7; const int greenLedPedestrian = 3; const int redLedPedestrian = 4; const int pushButton = 2; void setup() { pinMode(greenLedVehicle, OUTPUT); pinMode(yellowLedVehicle, OUTPUT); pinMode(redLedVehicle, OUTPUT); pinMode(greenLedPedestrian, OUTPUT); pinMode(redLedPedestrian, OUTPUT); pinMode(pushButton, INPUT_PULLUP); digitalWrite(greenLedVehicle, HIGH); digitalWrite(redLedPedestrian, HIGH); } void loop() { if(digitalRead(pushButton) == LOW) { digitalWrite(greenLedVehicle, LOW); digitalWrite(yellowLedVehicle, HIGH); delay(2000); digitalWrite(yellowLedVehicle, LOW); digitalWrite(redLedVehicle, HIGH); delay(1000); digitalWrite(redLedPedestrian, LOW); digitalWrite(greenLedPedestrian, HIGH); delay(5000); digitalWrite(greenLedPedestrian, LOW); digitalWrite(redLedPedestrian, HIGH); delay(1000); digitalWrite(redLedVehicle, LOW); digitalWrite(greenLedVehicle, HIGH); } } | greenLedVehicle is initialised to be PIN 5 yellowLedVehicle is initialised to be PIN 6 redLedVehicle is initialised to be PIN 7 greenLedPedestrian is initialised to be PIN 3 redLedPedestrian is initialised to be PIN 4 pushButton is initialised to be PIN 2
Within void setup() pinMode(greenLedVehicle, OUTPUT); pinMode(yellowLedVehicle, OUTPUT); pinMode(redLedVehicle, OUTPUT); pinMode(greenLedPedestrian, OUTPUT); pinMode(redLedPedestrian, OUTPUT); : Establishes PINs 3, 4, 5, 6, 7 as output pinMode(pushButton, INPUT_PULLUP) : Establishes pushButton as input
digitalWrite(greenLedVehicle, HIGH); digitalWrite(redLedPedestrian, HIGH); : PINs 4 and 5 are always on before pushing the pushButton Within voidloop() if(digitalRead(pushButton) == LOW) : when the pushButton is pushed... digitalWrite(greenLedVehicle, LOW); digitalWrite(yellowLedVehicle, HIGH); delay(2000); : PIN 5 turns off, PIN 6 turns on and there will be a 2-second delay digitalWrite(yellowLedVehicle, LOW); digitalWrite(redLedVehicle, HIGH); delay(1000); : PIN 6 turns off, PIN 7 turns on and there will be a 1-second delay digitalWrite(redLedPedestrian, LOW); digitalWrite(greenLedPedestrian, HIGH); delay(5000); : PIN 4 turns off, PIN 3 turns on and there will be a 5-second delay digitalWrite(greenLedPedestrian, LOW); digitalWrite(redLedPedestrian, HIGH); delay(1000); : PIN 3 turns off, PIN 4 turns on and there will be a 1-second delay digitalWrite(redLedVehicle, LOW); digitalWrite(greenLedVehicle, HIGH); PIN 7 turns off and PIN 5 turns on |
2. Below are the hyperlink to the sources/references that I used to write the code/program.
Hyperlink
3. Below are the problems I have encountered and how I fixed them.
Completing this task was as easy as eating a cake as I didn't have to make any adjustments to the code which we copied. I just had to simply attach the wires to their respective pins and I just smiled as I was the first person in class to interface 5 LEDs.
4. Below is the short video as evidence that the code/program work.
Below is my Learning Reflection on the overall Arduino Programming activities.
Learning about Arduino programming was quite refreshing as I definitely didn't expect that we would be learning how to code and connect circuits in Chemical Engineering. However, in this current day and age, coding is a common skill amongst most youths entering the working world, especially engineers. Even though Arduino programming doesn't really us to write our own code, I still feel like it's a good introduction to understanding what coding is like.
In our first-ever tutorial on Arduino Programming, I recall that Jianye and I were completely dumbfounded as we did not understand anything that was going on. We just copy and paste whatever code we found in the learning package and one of the lights started blinking and our servo motor started moving. We already felt very accomplished after achieving such a feat by not understanding any of the codes.
However, when it came to completing the pre-practical, it really got me to understand what certain functions in the code actually meant. This helped me understand the basics of how a code should be formatted and how the initial parts of the code are the most critical as it sets all the components as inputs and outputs. Everything else in the void loop can be adjusted very easily as we are just changing how the inputs and outputs react at different times. The following are the basic codes required to create something:
int Loremipsum = 13; | sets Loremipsum to be PIN 13 |
voidsetup() | setup will run once the board in power or the reset button is pushed |
pinMode(Loremipsum, OUTPUT); | sets Loremipsum as output |
pinMode(pushButton, INPUT_PULLUP); | sets the pushButton as input |
voidloop() | loops the code |
digitalWrite(Loremipsum, HIGH); | sets the voltage to high, causing any LED connected to Loremipsum to light up |
digitalWrite(Loremipsum, LOW); | sets voltage to low, causing any LED connected to Loremipsum to turn off |
delay(1000); | creates a 1 second delay before the next line of code is carried out |
The project which I had the most fun with was programming songs into the code for the Arduino to make some noise. To be fair, most Arduino codes are readily available online which makes it such a beginner-friendly tool. It was also very fun to code the 3 LEDs, but since Jianye and I wanted to experiment a little, we attempted the Traffic Light project using 5 LEDs. It was so interesting to see how the code worked co-currently with the physical demonstration itself and how it actually reenacted an actual traffic light.
Overall, learning the very basics of Arduino Programming was quite fun and I enjoyed sharing this experience with my friends. I hope that with the current skills and knowledge that I have, I would be able to create a reasonable programme for my group's CO Detector to work in our CA2.
Comentarios